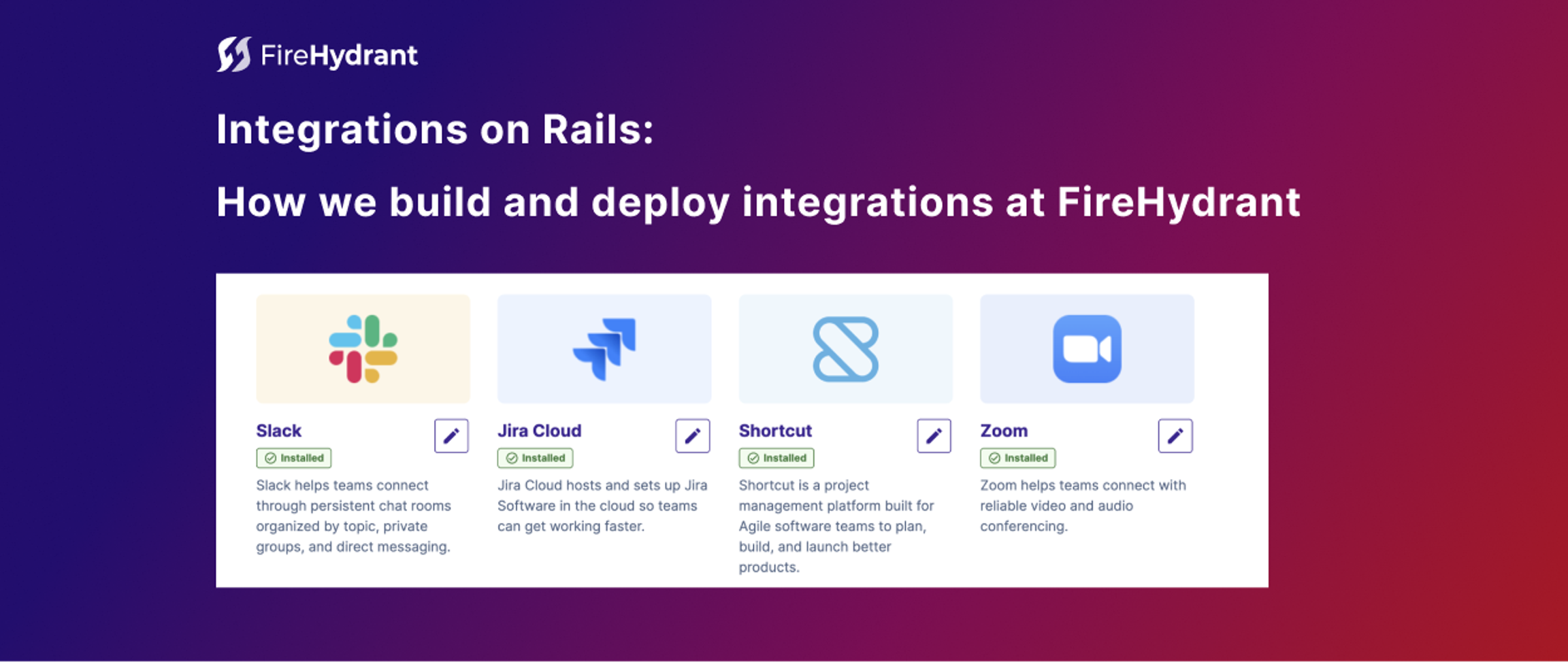
Integrations on Rails: How we build and deploy integrations at FireHydrant
This post explores how we built FireHydrant in a way that allows us to rapidly build and deploy integrations to help our product fit into responders’ workflows and not vice versa.
See FireHydrant in action
See how our end-to-end incident management platform can help your team respond to incidents faster and more effectively.