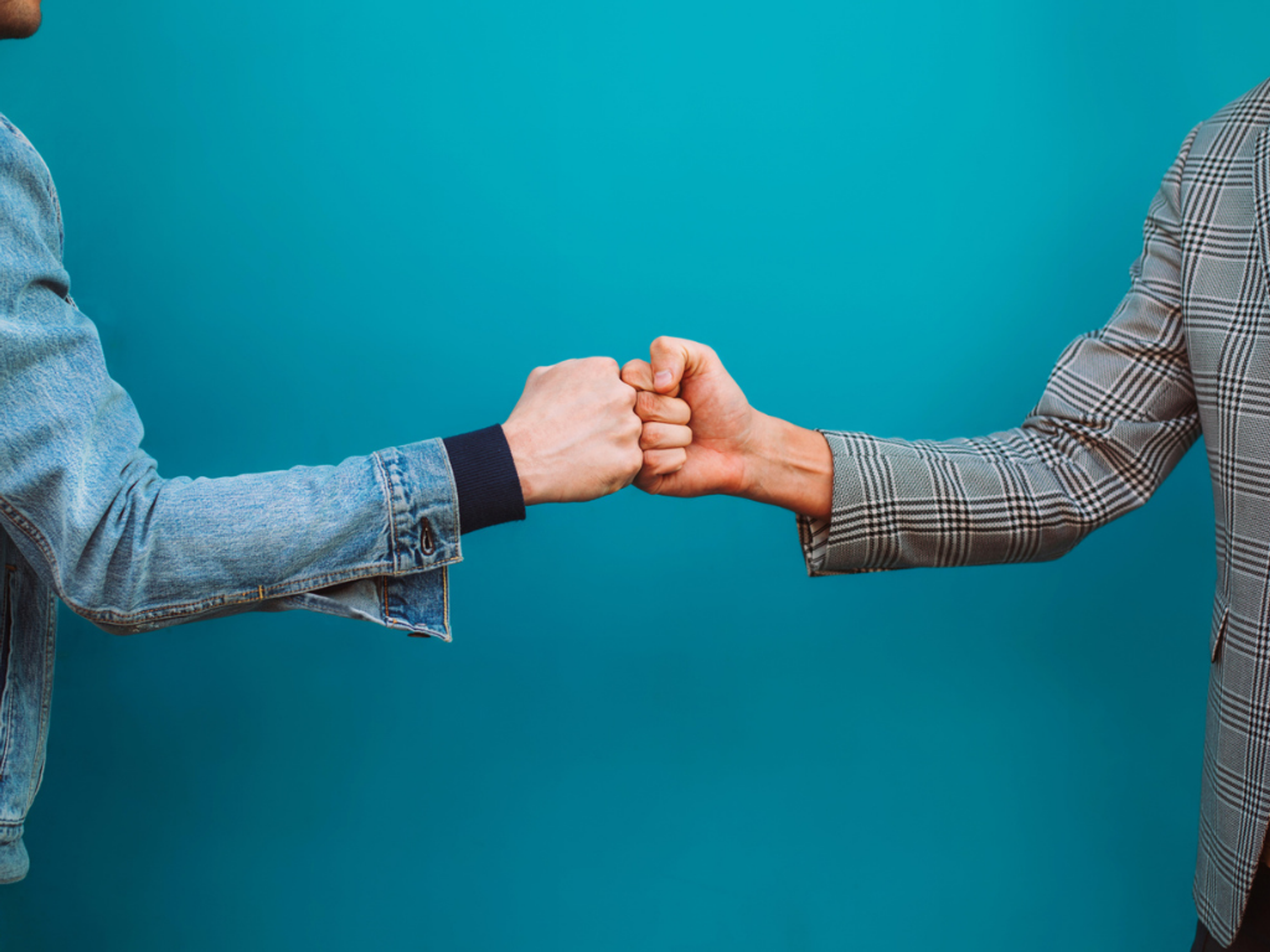
Working Together (but Separately) with MirageJS
We're using MirageJS to enable front-end and back-end teams to develop features asynchronously, without obstacles.
Note: This post was written by Hilary Beck, with much-appreciated help from front-end engineer Christine Yi.
Front-end development and APIs — you’ll rarely see one without the other, but they don’t always get up and running at once. Maybe you’re developing a completely new feature that relies on a nonexistent endpoint, or perhaps the endpoint is just out of date, missing key chunks of data. Front-end and back-end are being built simultaneously, but one isn’t available to the other just yet.
Often, front-end developers will hard-code values or write their own mock data sets as a quick fix. But that doesn’t give us a full view of the finished product: edge cases, changes to state, latency and request time. A lot of things can be missed! Even something as simple as coding for a string when the response is a number can derail all the work when the API is ready.
Our front-end guild recently decided to tackle this problem with MirageJS, a dynamic, relatively lightweight library that functions as a fake server. It runs in the client and handles network requests just as a real server would; this resolves the friction of having to wait for API work to be completed before you can work on a feature. It also enables rapid prototyping and quicker asynchronous development.
MirageJS factories favor framework-agnostic server-side models — structured, scaffolded-out data. When working on changes, back-end and front-end can get together at the discovery stage and decide what schema and API contracts will be. This easily translates to mocking out MirageJS’s data layer where the model definitions are made. Real interactions can be built by intercepting our local app’s network requests without a back-end API being present. There's no anticipating or hard-coding of expected data, and no need to modify UI code.
For instance, we recently redesigned our service catalog experience. We’ve already got some of the information we need in the existing endpoint, but it’s not quite as extensive as we'd like. In a review of design docs, we see new fields on our list page for values that we’re currently only getting as IDs. Both teams know what changes need to be made to the API, what the response should look like, and how it will be presented in the UI — and this is where MirageJS comes in.
In a new file MirageServer
, we first import createServer
and export a function makeServer
, which accepts an options arg with an environment key (Mirage has two: default development
and test
), that creates a new server:
The seeds
hook seeds our app with initial data so that we don’t start out empty. Within the routes()
hook, we can define our route handler and use this.get
to mock out our GET request.
We want to make sure Mirage is running when we render the services route, but not in production. Here’s where we call makeServer
:
When the new API is ready, it won’t require much to transition off Mirage. Remember, our requests are already pointing at the real API server; Mirage only intercepts them. Nothing has changed about our data fetch — we just need to delete the route from our mock server, remove the makeServer
call, and we’re good to ship.
Mirage’s test environment runs with zero delays, suppresses all logs, and ignores the seeds()
hook to prevent data leak. This makes it easy to test requests and responses in general without having to rely on configuring your local database.
With any project, issues of scaling and maintenance arise. As an organization grows and undergoes constant changes to the API, your MirageJS will need manual updates. You might have begun playing around with a one-off fake server on the component level, but it makes more sense to have a larger, centralized server like you would in production. For time-sensitive projects, the additional work is something to consider, but MirageJS does offer plenty of solutions for more complex configurations (mocking GUIDs, cookie responses) and ultimately behaves like any API contract would.
It’s given peace of mind to everyone, from front-end to back-end to design, while radically transforming our app. We can’t wait to see how it will help us collaborate even more.